Angular Signals: A Comprehensive Guide and Walkthrough
Exploring Angular Signals: Understanding Their Purpose, Pros, and Cons for Modern Development
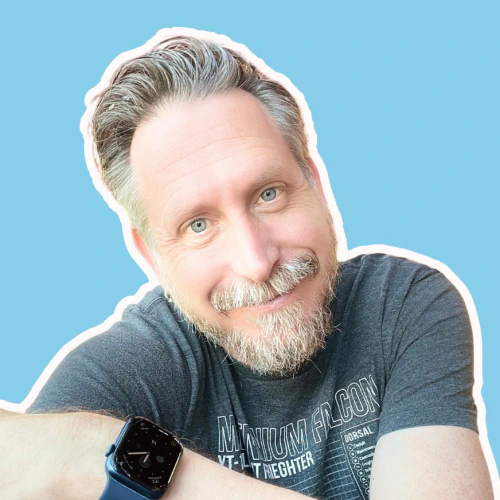
- Jay McBride
- 5 min read
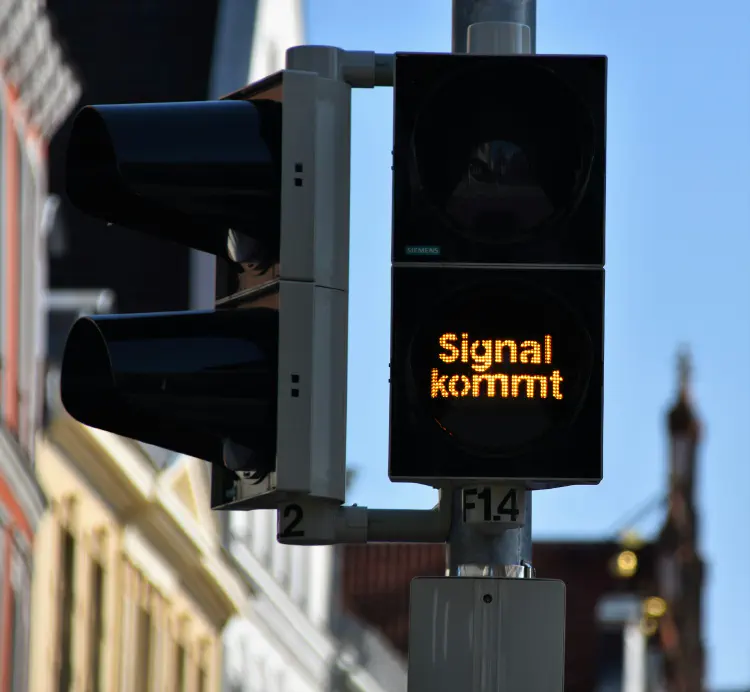
When Angular first introduced Signals, the buzz around it left many wondering if this could be a replacement for RxJS. However, after diving into Signals and understanding their role, it’s clear that Angular Signals serve a different but equally important purpose. They aim to simplify reactive programming, but they’re not here to replace RxJS. Instead, they provide a complementary approach to managing reactivity in Angular applications.
In this post, we’ll dive deep into what Angular Signals are, how they differ from RxJS, and whether they could ever replace it. We’ll also walk through how to use Angular Signals in your application and explore their pros and cons.
What Are Angular Signals?
At its core, Signals are a way to manage reactive state changes. Signals allow you to create a reactive source of truth in your Angular application and notify interested parties when that state changes. This is different from RxJS Observables, which are designed to handle streams of asynchronous data.
The goal of Signals is to simplify state management without the overhead and complexity that comes with RxJS. While RxJS handles complex streams and asynchronous flows, Signals focus on simple, synchronous reactivity.
Signals vs. RxJS: A Complement, Not a Replacement
Now, let’s address the elephant in the room: are Angular Signals meant to replace RxJS?
The short answer is no. RxJS is still the go-to for handling complex data streams and asynchronous operations, while Signals offer a simpler alternative for managing reactive state in a more synchronous, straightforward way.
One way to think about it is that Signals target more granular, UI-level changes, while RxJS is typically used for broader application-wide events, such as handling HTTP requests, user inputs, or complex event streams.
Let’s clarify this with a comparison:
- RxJS: Ideal for managing asynchronous tasks, like HTTP requests, real-time data, and streams.
- Signals: Perfect for handling local, synchronous state changes, such as form inputs, component reactivity, and smaller data updates.
When to use each:
If your application deals with constant, asynchronous data (such as a live data feed), RxJS is likely the better choice. If you’re managing more predictable state updates that don’t involve streams or async operations, Signals offer a lightweight, simple alternative.
The Purpose of Angular Signals
The main advantage of Signals is their simplicity. They’re designed to reduce boilerplate code and make managing local reactivity easier. Instead of dealing with subjects, subscriptions, and operators, as you would with RxJS, Signals offer a more declarative, less complex way to manage reactive state.
For example, imagine a scenario where you’re handling form inputs. Signals let you manage reactivity at the input level with minimal effort, while RxJS might add unnecessary complexity for such a simple task.
Pros and Cons of Angular Signals
Pros:
- Simplicity: Signals drastically reduce the complexity of managing reactive state, especially in cases where RxJS may feel like overkill.
- Performance: Since Signals are synchronous and more lightweight, they tend to offer better performance for smaller-scale reactivity.
- Declarative Syntax: The declarative approach makes it easier to reason about your reactive state, which simplifies debugging and maintenance.
- Focused Use: Perfect for local, synchronous state management that doesn’t need the complexity of streams.
Cons:
- Not a Full Replacement for RxJS: While simpler, Signals can’t replace RxJS for handling asynchronous data or complex workflows.
- Learning Curve: If you’re already familiar with RxJS, learning a new approach like Signals might add cognitive load.
- Limited Use Cases: Signals are great for managing local state but may not fit more advanced use cases involving asynchronous tasks.
Implementing Angular Signals: A Basic Walkthrough
Now, let’s walk through how you can implement Angular Signals in your application.
Step 1: Setup
First, ensure that your Angular app is set up and ready. You can generate a new Angular project if you don’t have one yet:
ng new angular-signals-demo
cd angular-signals-demo
Step 2: Create Your First Signal
In your Angular component, start by creating a Signal:
import { Component, Signal, signal } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<div>
<h1>{{ counter() }}</h1>
<button (click)="increment()">Increment</button>
</div>
`
})
export class AppComponent {
counter: Signal<number> = signal(0);
increment() {
this.counter.set(this.counter() + 1);
}
}
Here, we declare a Signal called counter
and initialize it with a value of 0. Each time the user clicks the button, the counter is incremented. Signals automatically update the DOM without needing complex subscriptions or change detection.
Step 3: Observe Changes with Computed Signals
Signals can also be composed into computed signals, allowing you to create reactive dependencies:
fullName: Signal<string> = computed(() => `${this.firstName()} ${this.lastName()}`);
This is useful for computed values that depend on other Signals and will update automatically when the dependencies change.
Step 4: Managing Component Reactivity
You can manage reactivity within components by simply updating the Signals. This reduces the boilerplate code and helps you focus on the UI logic rather than managing complex subscriptions:
isLoggedIn: Signal<boolean> = signal(false);
login() {
this.isLoggedIn.set(true);
}
Conclusion
Angular Signals offer a simpler, more declarative approach to managing reactive state in your applications. While they aren’t a replacement for RxJS, they provide a useful tool for handling local, synchronous state without the overhead of streams or subscriptions. By understanding the strengths and limitations of both Signals and RxJS, you can create a more efficient and maintainable Angular application.
What are your thoughts on Angular Signals? How do you see them fitting into your projects? Share your opinions and experiences in the comments below!