The Complete Guide to Performance Optimization in React Apps
Maximize Speed and Efficiency: Proven Tips for Optimizing React App Performance
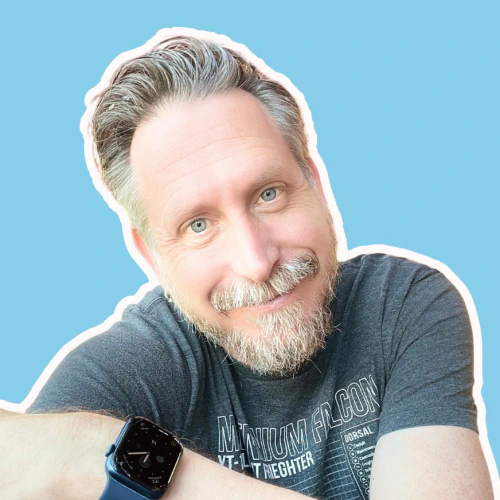
- Jay McBride
- 4 min read
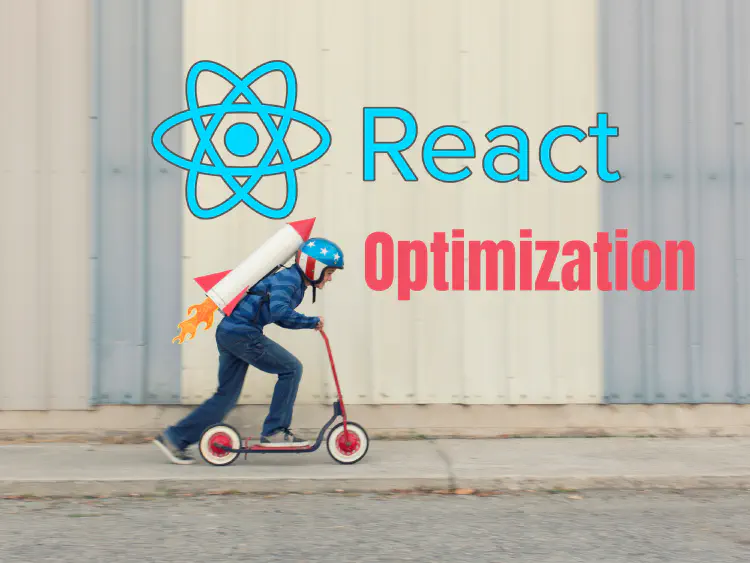
Performance is the backbone of any successful React application.
As developers, our mission isn’t just to build functional apps—it’s to deliver seamless, fast, and enjoyable experiences for users. Yet, achieving optimal performance can feel like navigating a maze of tools, techniques, and trade-offs.
In this guide, we’ll break down actionable strategies to optimize your React app, ensuring it’s both efficient and delightful to use.
1. Common React Performance Bottlenecks
Before diving into solutions, let’s identify the usual suspects behind sluggish React apps:
Unnecessary Re-renders: Components re-render even when there’s no need, wasting resources.
Large Bundle Sizes: Oversized JavaScript files lead to slow initial load times.
Inefficient State Management: Poorly organized state can cascade into performance issues.
Deep Component Trees: Complex structures make rendering and updates slower.
Understanding these bottlenecks helps focus your optimization efforts where they’ll have the most impact.
2. Optimize Component Rendering
Use React.memo
for Functional Components
Prevent unnecessary re-renders by wrapping components with React.memo
, which skips updates unless props change.
const ExpensiveComponent = React.memo(({ data }) => {
console.log('Rendering...');
return <div>{data}</div>;
});
Great for components receiving stable or rarely changing data.
Leverage useCallback
and useMemo
Use these hooks to memoize functions or computed values, reducing redundant calculations.
const memoizedCallback = useCallback(() => {
performHeavyOperation();
}, [dependency]);
const memoizedValue = useMemo(() => computeExpensiveValue(input), [input]);
Use shouldComponentUpdate
in Class Components
For class components, overriding the shouldComponentUpdate
lifecycle method gives precise control over re-renders.
shouldComponentUpdate(nextProps) {
return nextProps.value !== this.props.value;
}
3. Minimize Bundle Sizes with Code-Splitting
Large bundles slow down load times, especially on mobile networks. Code-splitting ensures users only download what they need for the current view.
import React, { Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
4. Implement Lazy Loading for Assets
Images, videos, and other assets can impact performance. Lazy loading ensures these elements load only when needed.
<img src="image.jpg" loading="lazy" alt="Lazy Loaded Image" />
For advanced use, consider libraries like react-lazy-load-image-component
.
5. Optimize State Management
Don’t Overuse Global State
While tools like Redux and Context API are powerful, they’re best reserved for truly global data. For local state, stick to useState
or useReducer
.
Use useReducer
for Complex Logic
When managing intricate state logic, useReducer
provides better organization and scalability.
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
default:
return state;
}
}
const [state, dispatch] = useReducer(reducer, { count: 0 });
6. Throttle and Debounce User Events
Frequent events like typing or scrolling can flood your app with updates. Debounce or throttle these events to control their frequency.
import { debounce } from 'lodash';
const handleInputChange = debounce((value) => {
console.log(value);
}, 300);
7. Improve Reconciliation Efficiency
Use Proper Keys in Lists
React uses key
props to track changes in lists. Ensure keys are unique and consistent.
{items.map((item) => (
<div key={item.id}>{item.name}</div>
))}
Avoid Excessive Wrappers
Use React.Fragment
instead of unnecessary <div>
wrappers to reduce DOM complexity.
<>
<h1>Title</h1>
<p>Content</p>
</>
8. Analyze and Fix Bottlenecks with React Profiler
The React Profiler provides insights into rendering performance, helping you pinpoint bottlenecks.
import { Profiler } from 'react';
<Profiler
id="MyComponent"
onRender={(id, phase, actualDuration) => {
console.log({ id, phase, actualDuration });
}}
>
<MyComponent />
</Profiler>
9. Audit Dependencies and Assets
Use tools like Bundlephobia to analyze the size of third-party libraries and find lighter alternatives. Removing unnecessary dependencies keeps your app lean.
10. Fine-Tune Your Production Build
Enable Production Mode
Ensure React is running in production mode for optimal performance.
NODE_ENV=production
Minify and Compress Assets
Tools like Webpack or Vite can reduce asset sizes for faster load times.
11. Consider Server-Side Rendering (SSR) or Static Site Generation (SSG)
Frameworks like Next.js or Gatsby offer SSR and SSG, which improve initial load times and SEO by pre-rendering content on the server.
12. Styling Optimization
Eliminate Unused CSS
Use tools like PurgeCSS to remove unused CSS, reducing stylesheet size.
Leverage Utility-First CSS Frameworks
Tools like Tailwind CSS streamline styling workflows, minimizing CSS bloat.
13. Improve Accessibility
Performance isn’t just about speed—it’s about usability.
Ensure accessible designs to provide a better experience for all users.
Conclusion: Performance is a Process
Optimizing a React app is an ongoing journey. Start small—focus on one or two strategies, measure their impact, and iterate. From reducing unnecessary renders to lazy loading assets, every step you take improves the user experience.
What are your favorite optimization strategies for React apps? Let’s discuss in the comments—I’d love to hear your ideas and challenges!